Breaking out of nested loops in Go involves using control flow mechanisms to exit from multiple nested loop levels prematurely based on certain conditions. Go does not have built-in support for labeled break statements like some other languages, but there are alternative approaches to achieve this.
One common technique is to use a boolean flag variable that controls the outer loop execution based on an inner loop condition. By setting the flag when the desired condition is met in the inner loop, the outer loop can check this flag and break out accordingly.
Another approach involves restructuring the nested loops into separate functions and using return statements to exit from the entire loop structure based on specific conditions.
Use Case 1:
import "fmt"
func main() {
var a int = 1
for a < 10{
fmt.Print("Value of a is ",a,"\n")
a++;
if a > 5{
/* terminate the loop using break statement */
break;
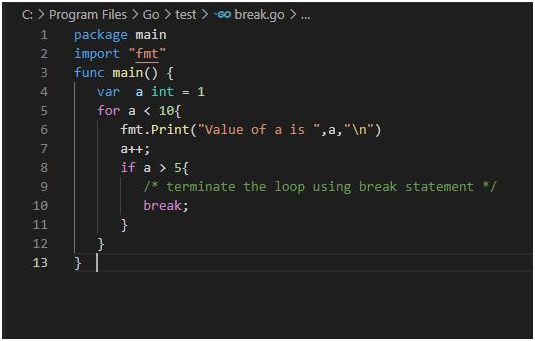
Output:
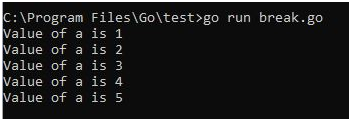
Use Case 2:
package main
import "fmt"
func main() {
var a int
var b int
for a = 1; a <= 3; a++ {
for b = 1; b <= 3; b++ {
fmt.Println(“Prwatech”)
if (a == 2 && b == 2) {
break;
fmt.Print(a, " ", b, "\n")
Output:
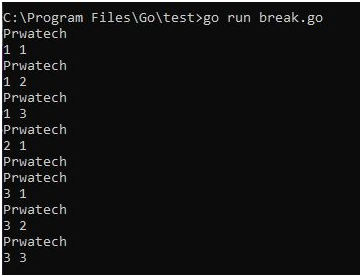
Use Case 3:
package main
import "fmt"
func main() {
var a int
var b int
for a = 1; a <= 3; a++ {
for b = 1; b <= 3; b++ {
if (a % 2 == 0) {
break;
fmt.Print(a, " ", b, "\n")
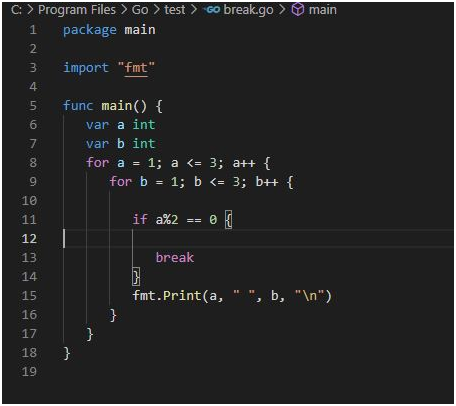
Output:
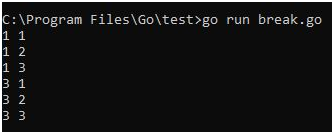