Introduction to Strings in Go
Strings in Go represent sequences of characters and are immutable, meaning their contents cannot be changed after creation. In Go, strings are represented as a sequence of bytes with each byte encoding a single character using UTF-8 encoding, which supports a wide range of international characters.
Go strings are commonly used for handling textual data such as names, messages, or file contents. They support various operations such as concatenation, substring extraction, length calculation, and comparison. Go's standard library provides a rich set of functions and methods for manipulating strings efficiently.
One notable feature of Go strings is their immutability, which ensures safety in concurrent programs where multiple goroutines may access the same string value simultaneously. Since strings are immutable, operations that appear to modify a string actually create and return new string values.
String is a sequence of characters.
Use Case 1:
package main
import (
"fmt"
"reflect"
)
var s string = "Hello World"
funcmain() {
fmt.Println(s)
fmt.Println(reflect.TypeOf(s))
}
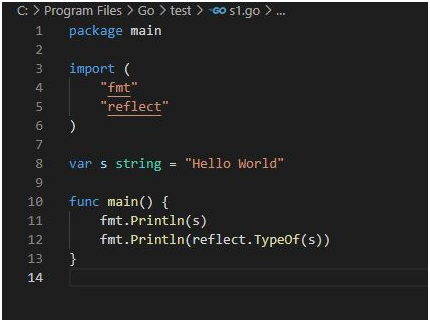
Output:

Use Case 2:
package main
import (
"fmt"
"strings"
)
funcmain() {
s := "Hello World"
fmt.Println(strings.HasSuffix(s, "IA"))
}
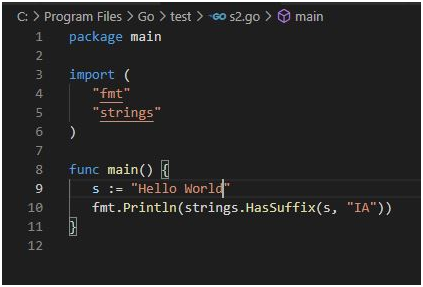
Output:
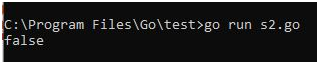
Use Case 3:
package main
import (
"fmt"
"strings"
)
funcmain() {
var str = "Hi "
fmt.Println(strings.Repeat(str, 4))
}
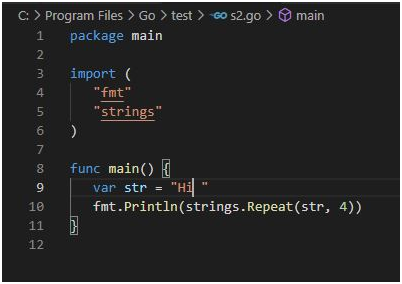
Output:
