A Guide to Scala Maps
Map:
Scala map is a collection of key/value pairs. Any value can be retrieved based on its key. Keys are unique in the Map, but values need not be unique. Maps are also called Hash tables. There are two kinds of Maps, the immutable and the mutable.
Scala maps support various operations such as adding new key-value pairs, updating values for existing keys, checking for the presence of keys, iterating over key-value pairs, and applying functional transformations using higher-order functions like map
, filter
, and fold
.
Scala also provides mutable map implementations (scala.collection.mutable.Map
) for scenarios where mutable key-value pairs are required. Understanding how to use maps effectively in Scala is essential for managing and manipulating associative data structures in functional and imperative programming paradigms. Maps offer a versatile and powerful way to organize and access data efficiently based on unique identifiers.
It is a universal concept, where it deals with Key-Value pair.
The fastest searching algorithm.
use case 1:
package Collection class Map1 { val
m
=
Map
(1 -> "Python", 2 -> "Scala", 3 -> "Hadoop") val
m1
=
Map
((91,"Ind"), (7,"USA"), (37,"USSR")) def courses(): Unit = {
println
(
m
.get(2))
println
(
m
.keys)
println
(
m
.values) } } object ME{ def main(args:Array[String]):Unit = { new Map1().courses()
println
("Maps is implemented") } }
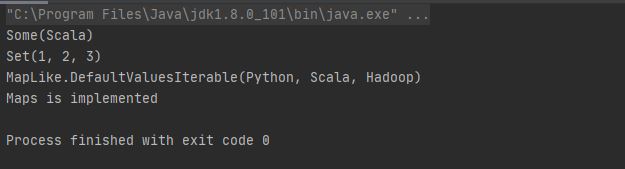
use case 2:
scala> val mapping = Map("Prwa" -> "Tech","Hadoop" -> "Developer","Spark" -> "Scala")
scala> val mapping = scala.collection.mutable.Map("Mysql" -> "S","Linux" -> "L","Python" -> "P")
1scala> val x = mapping("Mysql")
2scala> val x = mapping("Linux")
scala> val x = mapping("Python")
scala> for((s,l) <- mapping) yield(l,s)
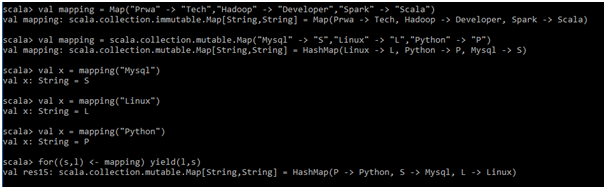
use case 3:
scala> val mapping = Map("Tom" -> "Hardy","Christian" -> "Bale")
scala> val mapping = scala.collection.mutable.Map("Leonardo" -> "D","Brad" -> "B")
1scala> val x = mapping("Tom")
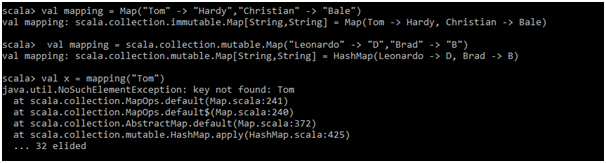
//Accessing maps
Contd..
#scala> val mapping = Map("Tom" -> "Hardy","Christian" -> "Bale")
scala> val mapping = scala.collection.mutable.Map("Leonardo" -> "D","Brad" -> "B")
scala> val x = mapping("Leonardo")
#Iterating Maps:
scala> for((d,b) <- mapping) yield(b,d)
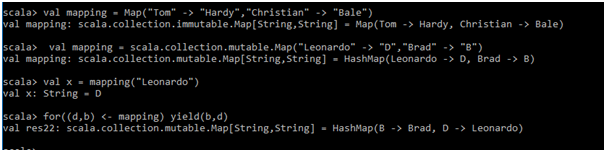
A Guide to Scala Maps