Abstract Classes in Scala
Scala abstract class concept is similar to java abstract class. it is created when there is a need to create a base class which requires constructor arguments.
Abstract classes in Scala provide a mechanism for defining partially implemented classes that cannot be instantiated directly but can be extended by concrete subclasses. An abstract class may contain abstract methods (methods without implementations) as well as non-abstract methods (methods with implementations).
To declare an abstract class in Scala, use the abstract
keyword before the class
keyword.
Abstract classes are useful for defining common behavior and characteristics that can be shared among multiple related classes while allowing specific details to be implemented in individual subclasses. They promote code reusability, encapsulation, and polymorphism in Scala applications.
Subclasses of an abstract class must implement all abstract methods defined in the abstract class to become concrete classes. This enables the concept of "contract-based programming," where abstract classes define contracts that subclasses must fulfill.
use case 1:
scala> abstract class Emp (name:String){
def id: Int
}
scala> class PrwatechEmp(name:String) extends Emp(name){
def id = name.hashCode
}
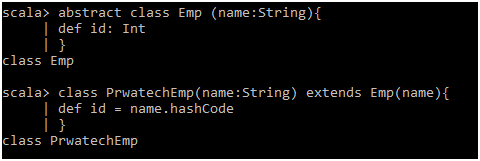
use case 2:
scala> abstract class eduprwa(name:String){
def id: Int
}
scala> class Prwa(name:String) extends Emp(name){
def id = name.hashCode
}
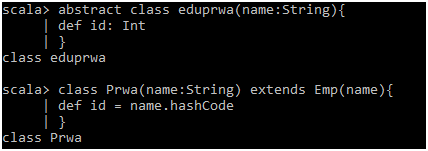
use case 3:
scala> abstract class BigData(name:String){
def id: Double
}
scala> class Training(name:String) extends Emp(name){
def id = name.hashCode
}
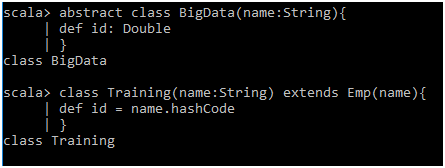