A Guide to Scala's Closures
Closure is a function in scala, it's return value depends on one or more variable which is declared outside the function.
use case 1:
object closure1 {
def main(args: Array[String]) {
println( "multiplier(1) value = " + multiplier(4) )
println( "multiplier(2) value = " + multiplier(5) )
}
var factor = 5
val multiplier = (i:Int) => i * factor
}
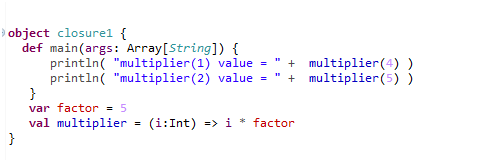
output

use case 2:
object closure2 {
// Main method
def main(args: Array[String])
{
println( "Final_Sum(1) value = " + sum(1))
2println( "Final_Sum(2) value = " + sum(2))
println( "Final_Sum(3) value = " + sum(3))
}
var a = 4
// define closure function
val sum = (b:Int) => b + a
}
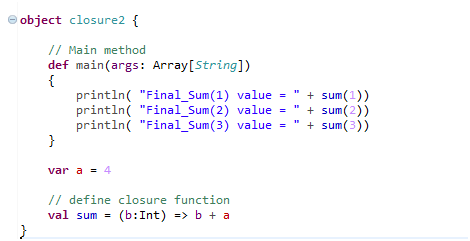
output
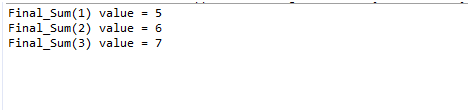
Here, In above program function sum is a closure function. var a = 4 is impure closure. the value of a is same and values of b is different.
use case 3:
object closure3 {
// Main method
def main(args: Array[String])
{
var employee = 30
// define closure function
val closure3 = (name: String) => println(s"Company name is $name"+
s" and total no. of employees are $employee")
closure3("prwatech")
}
}
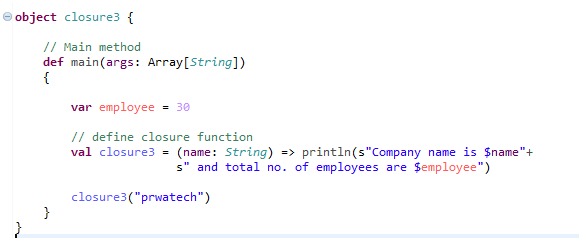
output

Here, In above example closure3 is a closure. var employee is mutable variable which can be change.