Higher Order Functions in Scala
In Scala, are the functions that take other functions as parameters or return a function as a result. This is because functions are first-class values in Scala. so we can say High order functions are the function for both methods and functions that can take functions as parameters or that return a function.
This powerful functional programming concept enables developers to write concise, expressive, and reusable code by treating functions as first-class citizens.
In Scala, functions are values that can be assign to variables, passe as arguments to other functions, or return from functions. This flexibility allows for the creation of higher order functions, which enhance code modularity and enable sophisticat abstractions.
Common examples of higher order functions in Scala include map
, filter
, reduce
, and fold
, which operate on collections and accept functions as arguments to perform transformations, filtering, and aggregations. By leveraging higher order functions, developers can abstract away common patterns and algorithms, making code more generic and reusable.
Use case 1
val salaries = Seq(20000, 70000, 40000)
val doubleSalary = (x: Int) => x * 2
val newSalaries = salaries.map(doubleSalary)
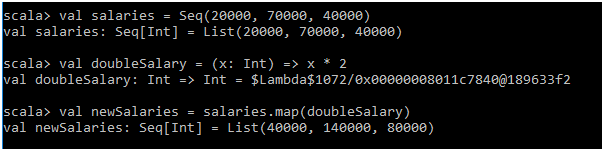
doubleSalary
is a function which takes a single Int, x
, and returns x * 2
. In general, the tuple on the left of the arrow =>
is a parameter list and the value of the expression on the right is what gets return. On line 3, the function doubleSalary
gets appli to each element in the list of salaries.
Use case 2
val salaries = Seq(20000, 70000, 40000)
val newSalaries = salaries.map(x => x * 2)
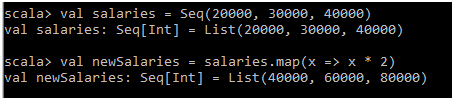
Use case 3:
// Scala program of higher order
// function
// Creating object
object prwatech {
// Main method
def main(arg:Array[String])
{
// Displays output by assigning
// value and calling functions
println(apply(format, 15))
}
// A higher order function
def apply(x: Double => String, y: Double) = x(y)
// Defining a function for
// the format and using a
// method toString()
def format[R](z: R) = "{" + z.toString() + "}"
}
output:
{15.0}