An Introduction to Scala Extractor Objects
In Scala Extractor is characterized as an item which has a technique named unapply as one of its part. This technique extracts an object and returns back the characteristics. The unapply technique reverses the development procedure of the apply method.
Scala extractor objects provide a mechanism for deconstructing objects in pattern matching contexts. An extractor object defines an unapply
method that extracts components from an object, enabling pattern matching to be used with custom data types. The unapply
method takes an object and returns an optional tuple of extracted values, allowing complex objects to be decomposed into simpler components.
By defining custom extractor objects, developers can extend pattern matching capabilities to user-defined classes and enable sophisticated matching logic.
use case 1:
scala> def apply(fname:String,lname:String)={
| fname+” “+lname
| }
scala> def unapply(str:String):Option[(String,String)]={
val parts=str split ” “
if(parts.length==2){
Some(parts(0),parts(1))
}else{
| None
}
| }
scala> apply(“Mark”,”Zuckerberg”)
2scala> unapply(“Mark Zuckerberg”)
scala> unapply(“MarkZuckerberg”)
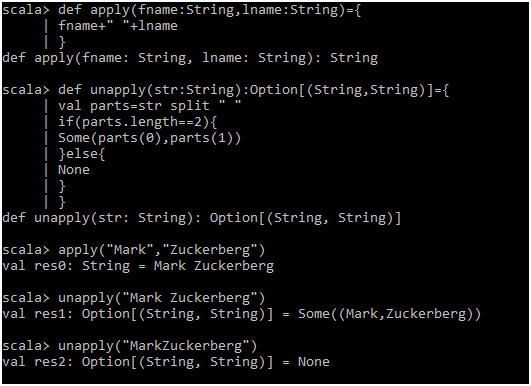
use case 2:
// Creating object
object prwatech {
// Main method
def main(args: Array[String])
{
// Assigning value to the
// object
val x = prwatech(50)
// Displays output of the
// Apply method
println(x)
// Applying pattern matching
x match
{
// unapply method is called
case prwatech(y) => println("The value is: "+y)
case _ => println("Can't be evaluated")
}
}
// Defining apply method
def apply(x: Double) = x / 5
// Defining unapply method
def unapply(z: Double): Option[Double] =
if (z % 5 == 0)
{
Some(z/5)
}
else None
}
output:
10.0
The value is: 2.0
use case 3:
// Scala program of extractors
to return a Boolean type
// Main method
// Creating object
object PRWATECH
{
def main(args: Array[String])
{
// Defining unapply method
def unapply(x:Int): Boolean = {
if (x % 5 == 0)
{
true
}
else
false
}
// Displays output in Boolean type
println ("Unapply method returns : " + unapply(16))
println ("Unapply method returns : " + unapply(35))
}
}
output:
Unapply method returns : false
Unapply method returns : true
An Introduction to Scala Extractor Objects