What is the apply function in Scala
In Scala, the apply
function is a special method defined in companion objects of classes or in standalone objects. The primary purpose of the apply
function is to provide a convenient way to create new instances of a class or invoke an object as if it were a function.
When you define an apply
method in a companion object, you can use it to construct instances of the corresponding class without explicitly calling the new
keyword. This allows for a more concise and readable syntax when creating instances. For example:
In this example, the apply
method in the Person
companion object enables us to create a new Person
instance simply by calling Person("Alice", 30)
, which internally delegates to Person.apply("Alice", 30)
.
In Scala the apply() method is used to select an element present in the list by its index position.
use case 1:
scala> object prwa{
def apply(name: String): String={
“Hello %s”.format(name)
}
}
object prwa
scala> prwa.apply(“prava”)
1scala> prwa(“binoj”)
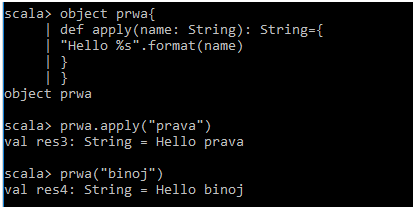
use case 2:
scala> class Prwatech {
private val elements = Array(“spark”,”scala”,”mysql”)
def apply(index:Int) = if(index<elements.length) elements(index) else “No element found”
}
class Prwatech
scala> val Prwatech = new Prwatech
2scala> println(Prwatech(1))
scala> println(Prwatech(0))
4scala> println(Prwatech(2))
scala> println(Prwatech(3))
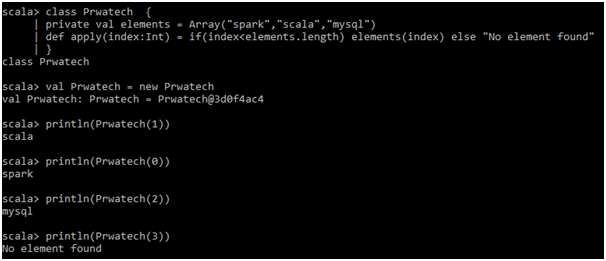
use case 3:
Scala> object prwatech{
def apply(name: String): String={
“My Name is %s”.format(name)
}
}
object prwatech
scala> prwatech.apply(“Mark”)
scala> prwatech.apply(“Steve”)
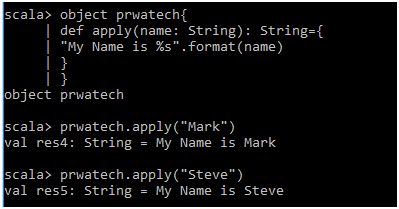
What is the apply function in Scala