Exception Handling in Scala
A Exception Handling in Scala is same as Java Exception Handling. In scala method can be terminated instead of returning by throwing an exception.
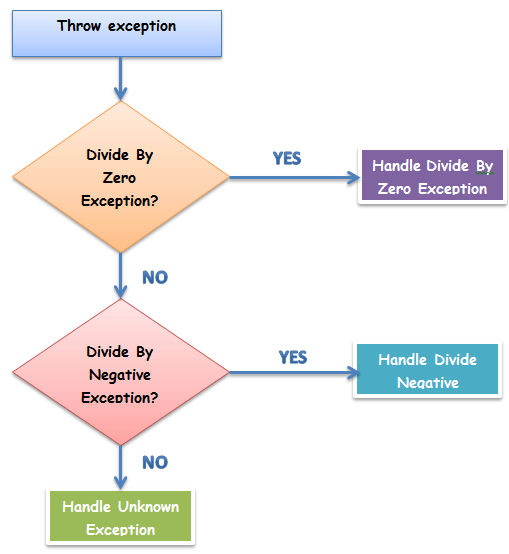
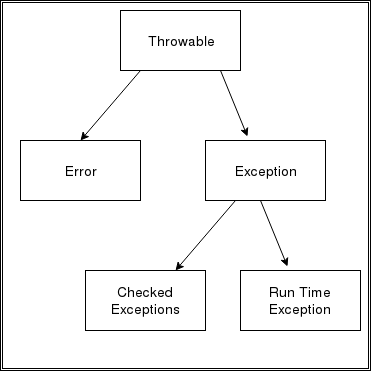
Use case 1 : try and catch
import java.io.FileReader
import java.io.FileNotFoundException
import java.io.IOException
object exceptioncatching {
def main(args: Array[String]) {
try {
val f = new FileReader(“input.txt”)
} catch {
case ex: FileNotFoundException =>{
println(“Missing file exception”)
}
case ex: IOException => {
println(“IO Exception”)
}
}
}
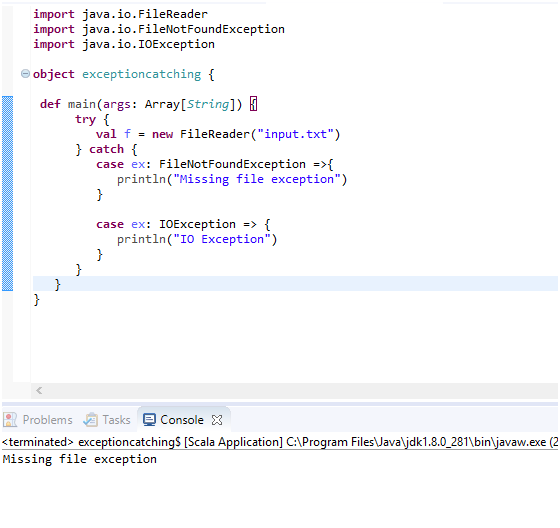
Use case 2 : finally
import java.io.FileReader
import java.io.FileNotFoundException
import java.io.IOException
object finalexception {
//main function
def main(args: Array[String]) {
try {
val f = new FileReader(“Read.txt”)
} catch {
case ex: FileNotFoundException => {
println(“Missing file exception”)
}
case ex: IOException => {
println(“IO Exception”)
}
} finally {
println(“Exiting finally…”)
}
}
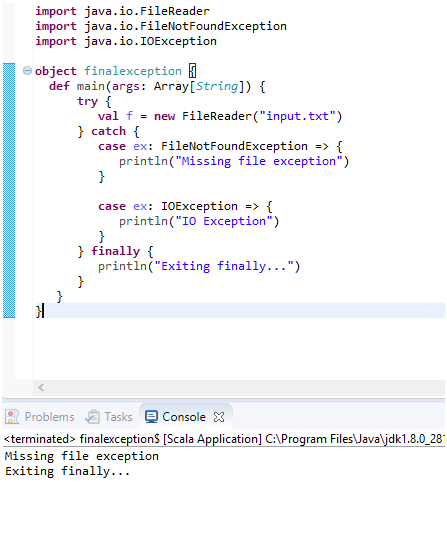
use case 3: Throw
object throwobj {
def validate(article:Int)=
{
// Using throw keyword
if(article < 20)
throw new ArithmeticException(“You are not eligible for internship”)
else println(“You are eligible “)
}
// Main method
def main(args: Array[String])
{
validate(22)
}
}
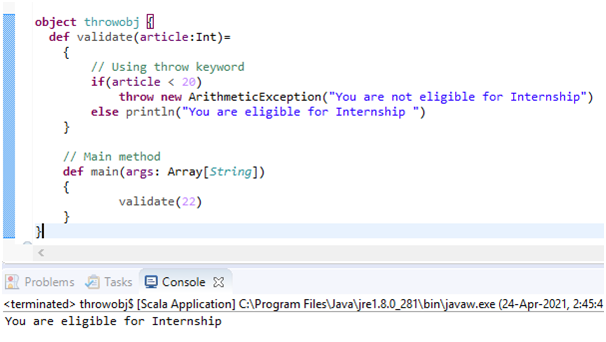