Golang Switch Case Conditional Statements
In Go (Golang), the switch statement provides a flexible and concise way to implement conditional branching based on the value of an expression. Unlike traditional switch statements in other languages, Go's switch statement does not require explicit break statements; instead, it automatically breaks at the end of each case block unless followed by a fallthrough keyword.
The switch statement in Go can be used to evaluate various types of expressions, including integers, strings, and other comparable types. It supports multiple comma-separated values in each case, allowing for concise matching against multiple conditions.
Go's switch statement also supports optional initialization statements that are scoped to the switch block, enabling the use of short-lived variables specific to each case. Additionally, the switch statement can be used without an expression, effectively creating an alternative to if-else chains based on boolean conditions.
Golang switch statements are similar to jump statements in algorithms.
Use Case 1:
package main
import "fmt"
func main() {
fmt.Print("Enter Number: ")
var i int
fmt.Scanln(&i)
switch (i) {
case 1:
fmt.Print("the value is 10")
case 2:
fmt.Print("the value is 20")
case 3:
fmt.Print("the value is 30")
case 4:
fmt.Print("the value is 40")
default:
fmt.Print(" It is not in bound ")
}
}
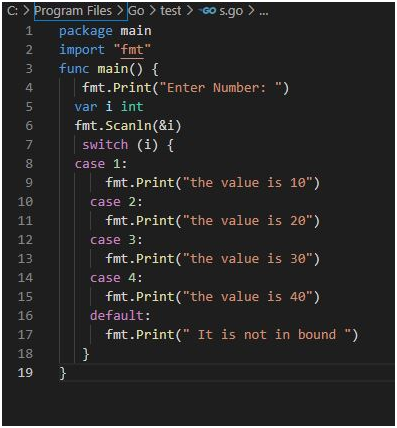
Output:

Use Case 2:
package main
import "fmt"
func main() {
switch day:=4; day{
case 1:
fmt.Println("Monday")
case 2:
fmt.Println("Tuesday")
case 3:
fmt.Println("Wednesday")
case 4:
fmt.Println("Thursday")
case 5:
fmt.Println("Friday")
case 6:
fmt.Println("Saturday")
case 7:
fmt.Println("Sunday")
default:
fmt.Println("Invalid")
}
}
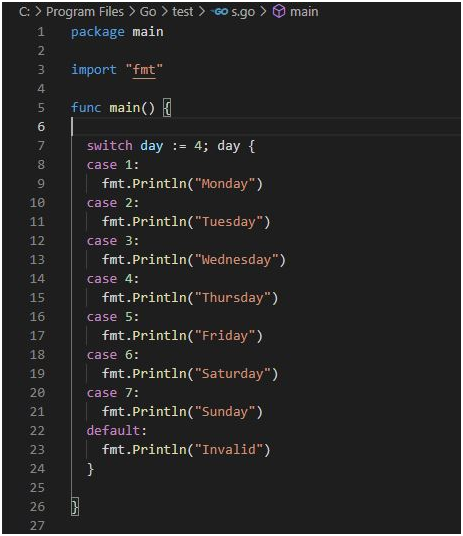
Output:

Use Case 3:
package main
import "fmt"
func main() {
var value string = "five"
switch value {
case "one":
fmt.Println("C#")
case "two", "three":
fmt.Println("Go")
case "four", "five", "six":
fmt.Println("Java")
}
}
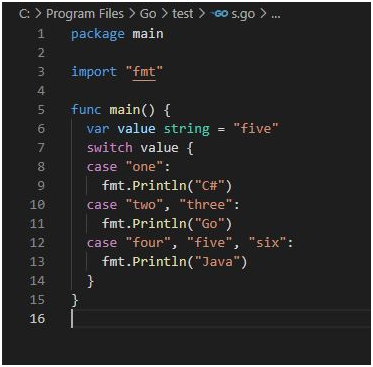
Output:
