Switch Case with Break in For Loop in Golang
In Go (or Golang), the switch
statement provides a flexible and concise way to evaluate multiple conditions and execute different blocks of code based on the value of an expression. When combined with a for
loop, the switch
statement allows for dynamic decision-making within iterative processes.
In Go, unlike some other languages, the switch
statement automatically breaks after executing a case block, meaning that there’s no need for an explicit break
statement at the end of each case block. This behavior prevents fall-through, where execution would continue to the next case block without a break statement.
When using a switch
statement inside a for
loop in Go, each iteration of the loop evaluates the expression provided to the switch
statement. Based on the evaluated value, the corresponding case block is executed, and control returns to the loop for the next iteration.
Break is used to exit from the loop.
Use Case 1:
package main
import “fmt”
func main() {
var a int = 1
for a < 10{
fmt.Print(“Value of a is “,a,”\n”)
a++;
if a > 5{
/* terminate the loop using break statement */
break;
Output:
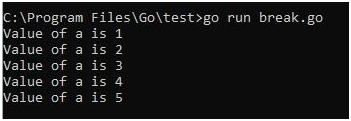
Use Case 2:
package main
import “fmt”
func main() {
var a int
var b int
for a = 1; a <= 3; a++ {
for b = 1; b <= 3; b++ {
fmt.Println(“Prwatech”)
if (a == 2 && b == 2) {
break;
fmt.Print(a, ” “, b, “\n”)
Output:
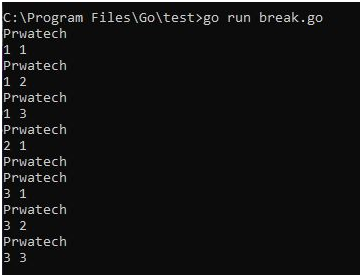
Use Case 3:
package main
import “fmt”
func main() {
var a int
var b int
for a = 1; a <= 3; a++ {
for b = 1; b <= 3; b++ {
if (a % 2 == 0) {
break;
fmt.Print(a, ” “, b, “\n”)
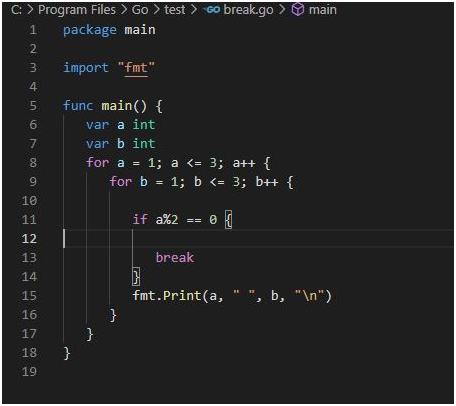
Output:
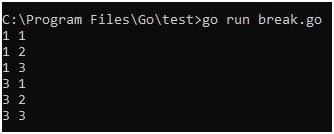