Best Practices for Interfaces in Go
In Go, interfaces play a crucial role in defining behavior and promoting flexibility in code design. Interfaces enable polymorphism by allowing different types to satisfy the same interface contract, facilitating code reuse and decoupling implementations from specific types.
To adhere to best practices when using interfaces in Go:
-
Keep Interfaces Small and Focused: Define interfaces with a small number of methods that capture a specific behavior or capability. This promotes clarity and makes interfaces easier to implement.
-
Use Interfaces Sparingly: Favor concrete types over interfaces unless there is a clear benefit to using an interface. Overusing interfaces can lead to unnecessary abstraction and increased complexity.
-
Name Interfaces Descriptively: Use meaningful and descriptive names for interfaces that reflect their purpose and functionality. This enhances code readability and comprehension.
Interface is a protocol – a contract. It only describes the expect behavior, It is an abstract type.
Use Case 1:
Program to print value through interface.
package main
import “fmt”
funcmyfunc(a interface{}) {
v := a.(string)
fmt.Println(“value :”, v)
}
funcmain() {
var v interface {
} = “Prwatech”
myfunc(v)
}
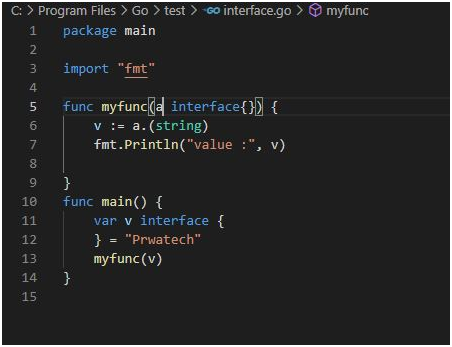
Output :

Use Case 2:
Program for geometric shapes.
package main
import (
“fmt”
“math”
)
type geometry interface {
area() float64
perim() float64
}
type rect struct {
width, height float64
}
type circle struct {
radius float64
}
func (r rect) area() float64 {
return r.width * r.height
}
func (r rect) perim() float64 {
return 2*r.width + 2*r.height
}
func (c circle) area() float64 {
return math.Pi * c.radius * c.radius
}
func (c circle) perim() float64 {
return 2 * math.Pi * c.radius
}
funcmeasure(g geometry) {
fmt.Println(g)
fmt.Println(g.area())
fmt.Println(g.perim())
}
funcmain() {
r := rect{width: 3, height: 4}
c := circle{radius: 5}
measure(r)
measure(c)
}
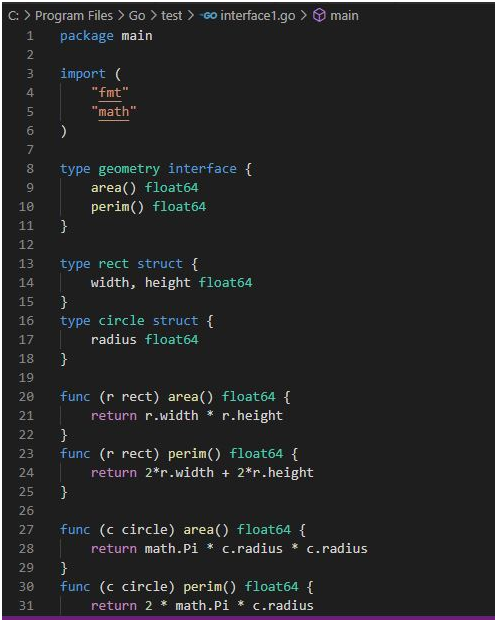
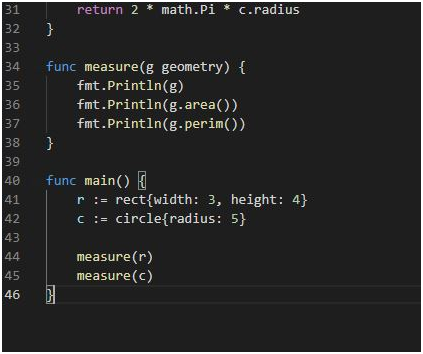
Output:
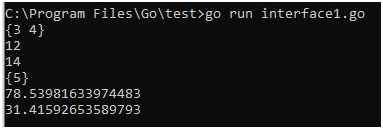
Use Case 3:
package main
import (
“fmt”
)
funcmyFunc(a interface{}) {
fmt.Println(a)
}
funcmain() {
var my_age int
my_age = 25
myFunc(my_age)
}
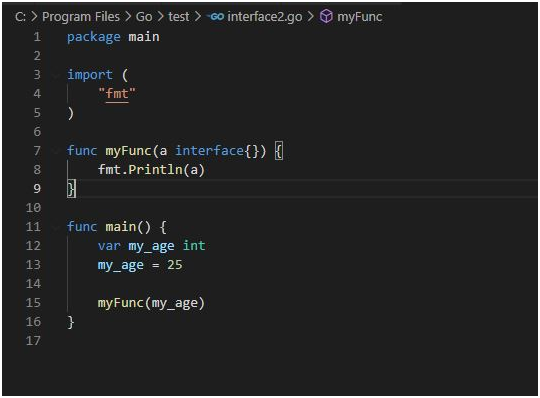
Output :
