Use cases of recursion in GoLang
Recursion is a programming technique where a function calls itself directly or indirectly to solve a problem by breaking it down into smaller, similar subproblems. In GoLang (Go), recursion is supported and can be employed for various use cases across software development.
One common use case of recursion in Go is in algorithms involving tree structures or hierarchical data. Recursive functions can efficiently traverse and manipulate tree-like data structures such as binary trees, linked lists, or directory structures.
Another use case is in implementing algorithms like factorial computation or Fibonacci sequence generation, where each step relies on the solution of a smaller subproblem. Recursion can elegantly express these algorithms by reducing complex problems into simpler repetitive tasks.
Recursion is a concept to calling a function within a function itself.
Use Case 1:
Finite Recursion : In finite function , the function stops at a finite number of steps.
Program – To print fibonacci series
package main
import “fmt”
func fib(i int) int {
if i == 0 {
return 0
}
if i == 1 {
return 1
}
return fib(i-1) + fib(i-2)
}
func main() {
var i int
for i = 0; i< 10; i++ {
fmt.Printf(” %d “, fib(i))
}
}
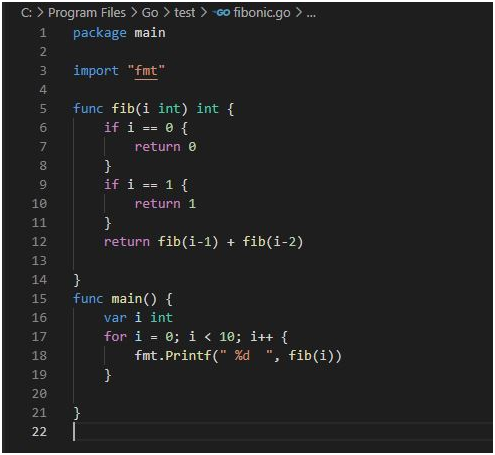
Output :

Use Case 2:
Program to print numbers by recursive function.
package main
import (
“fmt”
)
funcprintnum(n int) {
if n > 0 {
printnum(n – 1)
fmt.Println(n)
}
}
func main() {
printnum(5)
}
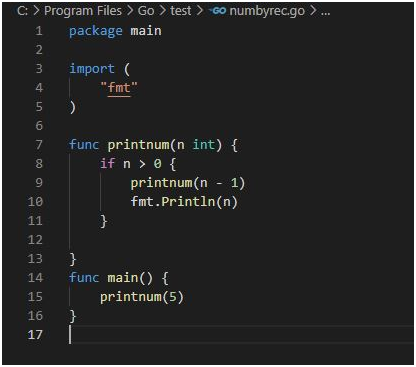
Output :
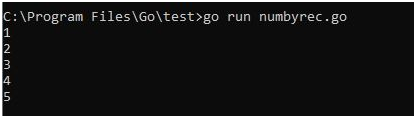
Use Case 3:
Infinte Recursion : In infinite recursion the function doesn’t stop calling itself, it runs infinite times.
package main
import (
“fmt”
)
func rec(){
fmt.Println(“Endless”)
rec()
}
func main() {
}
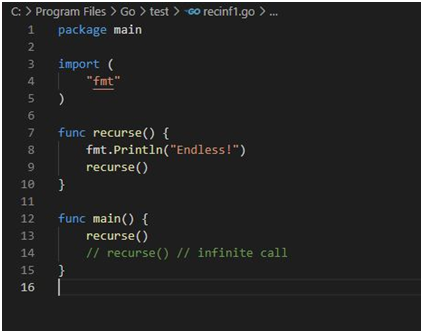
Output :
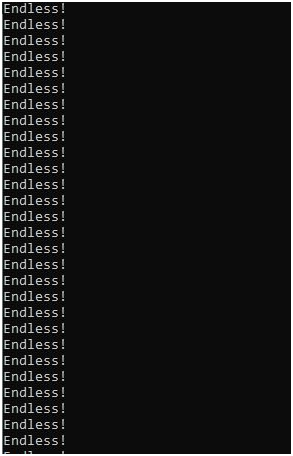