Use cases of functions in Golang
Functions in Go (Golang) are essential building blocks of software development, offering encapsulation, reusability, and modularity in code. They are used to define reusable blocks of logic that can be invoked multiple times with different inputs, enhancing code organization and readability.
One key use case of functions in Go is abstraction. By encapsulating specific tasks or behaviors into functions, developers can hide implementation details and expose only the necessary interfaces, promoting modular and maintainable code.
Functions also facilitate code reuse by allowing developers to define common operations as functions that can be invoked from multiple parts of the codebase. This promotes the “Don’t Repeat Yourself” (DRY) principle and reduces redundancy.
Function is a group of statements which is used to break large tasks into smaller tasks.
The Go standard libraries provides numerous built-in functions.
Defining a Functions
>> Func func_name ([Parameter_list]) [Return_type]
{
\\ body of a program
}
Use Case 1: Program to find maximum no.
package main
import “fmt”
func main() {
var a int = 100
1var b int = 211
var c int
c = max(a, b)
fmt.Println(“Max value is:”, c)
}
func max(a, b int) int {
if a > b {
return a
} else {
return b
}
}
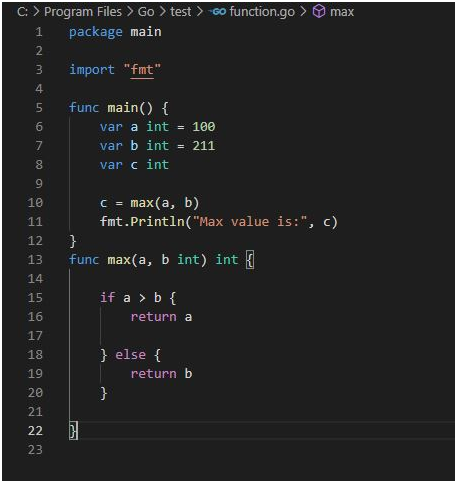
Output :

Use Case 2: Program to swap string
package main
import “fmt”
func swap(x, y string) (string, string) {
return y, x
}
func main() {
a, b := swap(“Prwatech”, “Bangalore”)
fmt.Println(a, b)
}
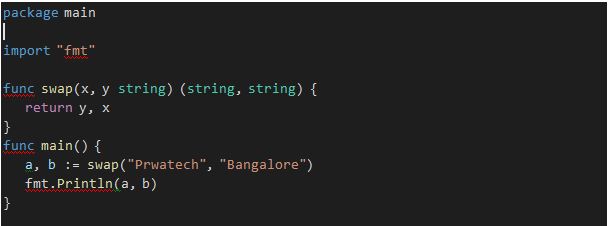
Output :

Use Case 3:
package main
import “fmt”
type Employee struct {
fname string
lname string
}
func (emp Employee) fullname(){
fmt.Println(emp.fname+” “+emp.lname)
}
func main() {
e1 := Employee{ “Alex”,”A”}
e1.fullname()
}
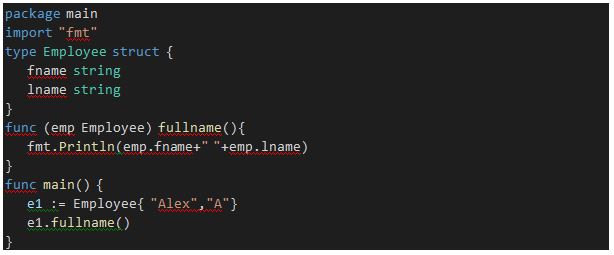
Output:
