Creating Maps in GoLang: Step-by-Step Tutorial
A Map is a type of data structure, it is a collection of unorder pairs of key-value. With the help of map we can easily do retrieve, update and insert operations.
Syntax:
var mymap map[keytype]valuetype
Use Case 1:
package main
import “fmt”
func main() {
var mymap map[int]int
if mymap == nil {
fmt.Println(“true”)
} else {
fmt.Println(“False”)
}
}
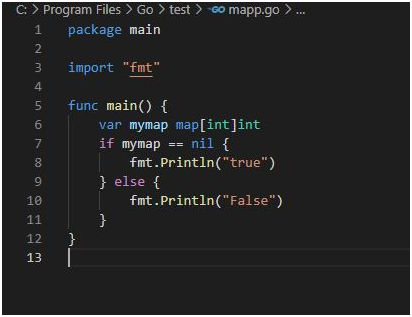
Output:

Use Case 2:
package main
import “fmt”
func main() {
var mymap map[int]int
if mymap == nil {
fmt.Println(“true”)
} else {
fmt.Println(“False”)
}
map2 := map[int]string{
10: “Prwatech”,11: “Bangalore”,
}
fmt.Println(“Map 2 value”, map2)
}
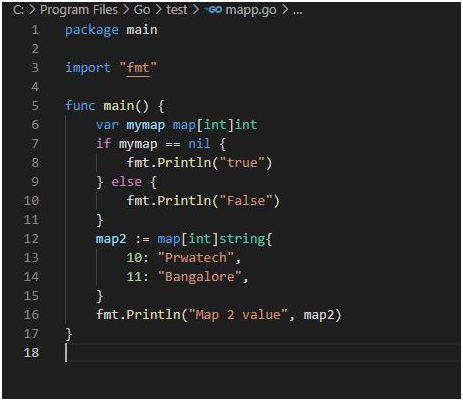
Output:

Use Case 3: Create a map using make() function.
package main
import “fmt”
func main() {
// Creating a map
// Using make() function
var map1 = make(map[int]string)
fmt.Println(map1)
// As we already know that make() function
/ always returns a map which is initialized
// So, we can add values in it
map1[1] = “Prwatech”
map1[2] = “Bangalore”
fmt.Println(map1)
}
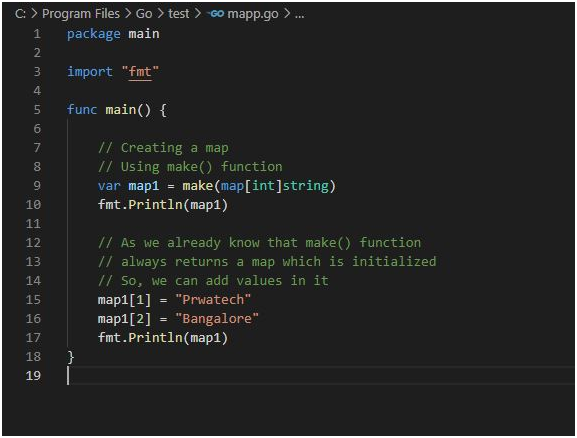
Output:
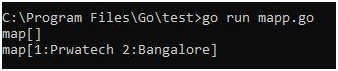
Use Case 4: Adding new key elements and update operation:
package main
import “fmt”
// Main function
func main() {
// Creating and initializing a map
m_a_p := map[int]string{
90: “Prwatech”,
91: “Bangalore”,
92: “Big Data”,
}
fmt.Println(“Original map: “, m_a_p)
// Adding new key-value pairs in the map
m_a_p[93] = “Python”
m_a_p[94] = “Golang”
fmt.Println(“Map after adding new key-value pair:\n”, m_a_p)
// Updating values of the map
m_a_p[93] = “Gcp”
fmt.Println(“\nMap after updating values of the map:\n”, m_a_p)
}
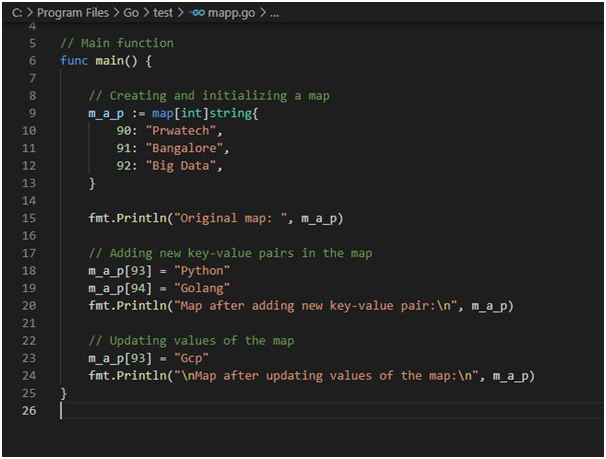
Output:
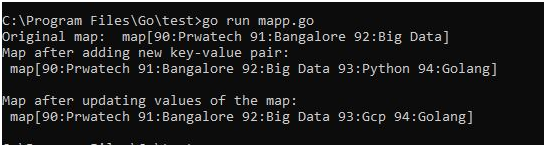
Use Case 5:Retrieving values with the help of keys:
package main
import “fmt”
// Main function
func main() {
// Creating and initializing a map
m_a_p := map[int]string{
90: “Red”,
91: “Yellow”,
92: “Orange”,
93: “Blue”,
94: “White”,
}
fmt.Println(“Original map: “, m_a_p)
// Retrieving values with the help of keys
value_1 := m_a_p[90]
value_2 := m_a_p[93]
fmt.Println(“Value of key[90]: “, value_1)
fmt.Println(“Value of key[93]: “, value_2)
}
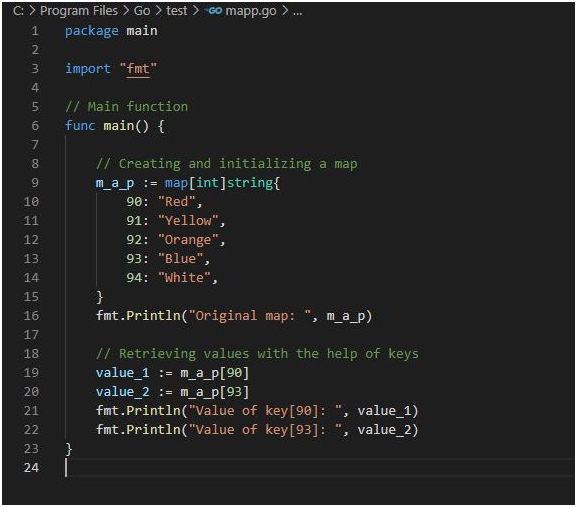
Output:

Use Case 6:Deleting keys
package main
import “fmt”
// Main function
func main() {
// Creating and initializing a map
m_a_p := map[int]string{
90: “Red”,
91: “Yellow”,
92: “Orange”,
93: “Blue”,
94: “White”,
}
fmt.Println(“Original map: “, m_a_p)
// Deleting keys
// Using delete function
delete(m_a_p, 90)
delete(m_a_p, 93)
fmt.Println(“Map after deletion: “, m_a_p)
}
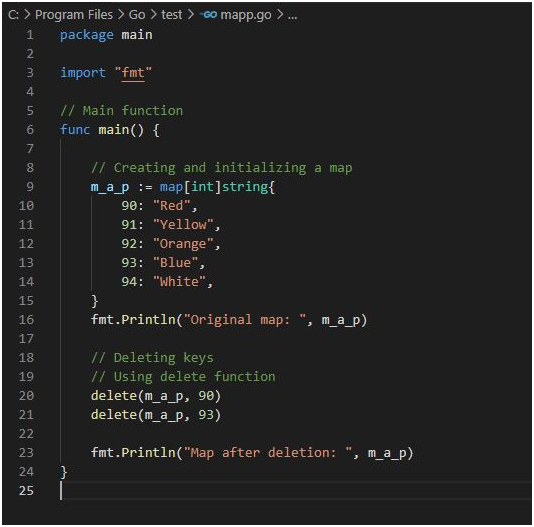
Output:
